Use razor sections in MVC4 to put javascript in its place
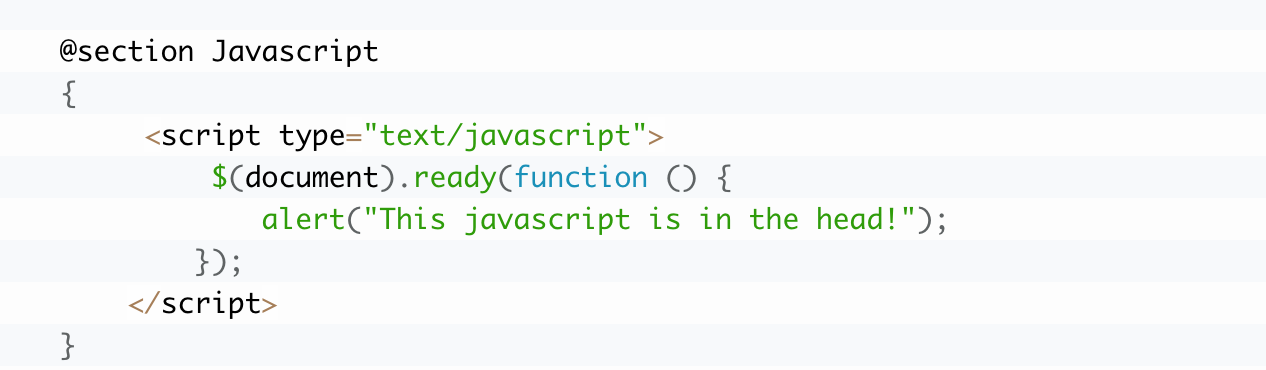
This is just a small tip, but one that I use in all of my MVC projects.
When you add javascript to a view (which I do quite often), it is rendered inside the page body, along with the rest of the view content. That's not best practice. Ideally, we want all javascript to be in the head of the page. Fortunately, MVC sections make this simple. In _Layout.cshtml add @RenderSection
<head>
<meta charset="utf-8" />
<title>@ViewBag.Title - My ASP.NET MVC Application</title>
[...links to script and CSS files...]
@RenderSection("JavaScript", required: false)
</head>
This tells the layout to look for a section named "JavaScript" in the view that's being rendered. Also note the "required: false". If this is not set or true, an error will be thrown if a view doesn't have that section.
In your view, wrap your Javascript in a section:
@section Javascript
{
<script type="text/javascript">
$(document).ready(function () {
alert("This javascript is in the head!");
});
</script>
}
That's it. Hope that helps make your code a little more elegant :)