Real Time Temperature and Humidity Graph with Tessel
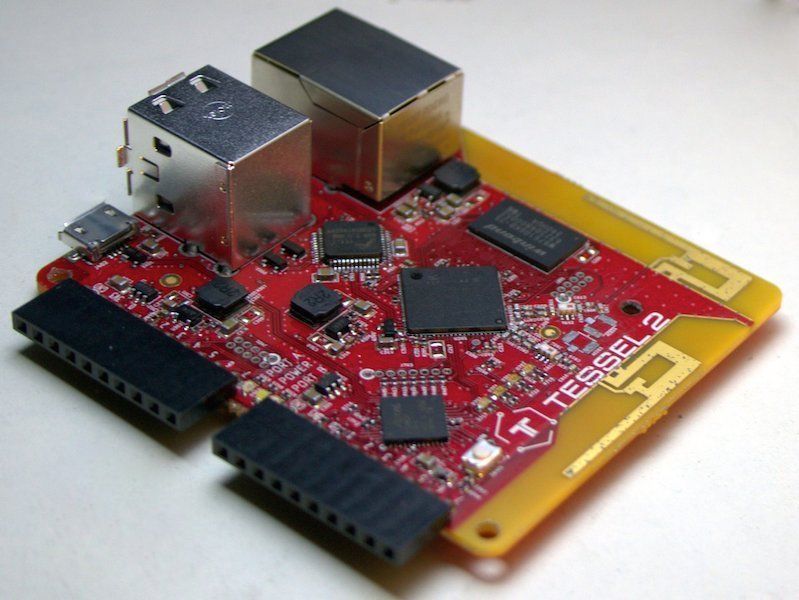
TL;DR: I built a weather station using a Tessel that posts data to Firebase. I'm displaying the temperature/humidity in a live-updating highchart that you can see here. Big "but": The Wifi on the Tessel isn't that reliable and locks up fairly frequently. Sometimes after 30 minutes, sometimes after several hours. The only way to fix that is to reboot the Tessel.
So, have you heard of this language called JavaScript? I hear that it's everywhere now. I know what you think: "Everywhere? What about microcontrollers? Surely nobody is crazy enough to use JavaScript as a language for programming microcontrollers".
Well turns out the good folks at Tessel are. I just got a Tessel 1 with the climate and accelerometer modules. Tessel is a little more plug and play than other microcontrollers in that there are a bunch of preconfigured modules that you really just have to plug into the board, install the library via npm (I know, right?) and off you go. Here's a code example for logging the temperature/humidity readings to the console every 10 seconds:
var tessel = require('tessel');
var climatelib = require('climate-si7020');
var climate = climatelib.use(tessel.port['A']);
climate.on('ready', function() {
console.log('Connected to si7020');
// Loop forever
setImmediate(function loop() {
climate.readTemperature('f', function(err, temp) {
climate.readHumidity(function(err, humid) {
console.log('Degrees:', temp.toFixed(4) + 'F', 'Humidity:', humid.toFixed(4) + '%RH');
setTimeout(loop, 10000);
});
});
});
});
```
Yes, that's all there is to it.
But wait, Tessel comes with on-board Wifi, so it's probably super easy to put this climate data somewhere in the cloud so I can then keep an eye on the coziness of my office, right? Right!
Set up Tessel's Wifi in the Terminal:
`tessel wifi -n YOURSSID -p PASSWORDHERE`
Now we just need to write a little piece of code that posts the temperature data to some kind of cloud storage. I'm using Firebase here, just because I've been wanting to check it out. Because we're just writing JavaScript and Tessel runs node, we can simply require the https module and write a simple POST:
````prettyprint lang-js
var https = require('https');
function post(temp, humid) {
var req = https.request({
port: 443,
method: 'POST',
hostname: 'YOURAPP.firebaseio.com',
path: '/climate.json',
headers: {
Host: 'YOURAPP.firebaseio.com',
'Accept': '*/*',
'User-Agent': 'tessel'
}
}, function(res) {
console.log('statusCode: ', res.statusCode);
});
req.write('{"datetime": ' + new Date().getTime() + ', "temp": ' + temp + ', "humid": ' + humid + '}');
req.write('\r\n');
req.end();
req.on('error', function(e) {
console.error(e);
});
}
```
We can now call this post-method from the loop in which we're reading the climate data:
````prettyprint lang-js
climate.readHumidity(function(err, humid) {
post(temp.toFixed(4), humid.toFixed(4));
setTimeout(loop, 10000);
});
```
OK, that took about 5 minutes.
Now, let's visualize this. Firebase with it's streaming API is perfect for a live-updating graph such as highcharts. Here's the relevant code to set up a streaming connection to Firebase and update the data points in a graph whenever new data comes in:
````prettyprint lang-js
var fb = new Firebase('https://FIREBASEACCOUNT.firebaseio.com/climate');
fb.on('child_added', function(data) {
var dataVal = data.val();
// add temperature point to series 0, don't redraw yet (false)
$('#container').highcharts().series[0].addPoint({
x: new Date(dataVal.datetime),
y: dataVal.temp
}, false);
// add humidity point to series 0, don't redraw yet (false)
$('#container').highcharts().series[1].addPoint({
x: new Date(dataVal.datetime),
y: dataVal.humid
}, false);
// added points; redraw
$('#container').highcharts().redraw();
});
```
That code gives us the following live-updating graph:
<a name="graph"></a>
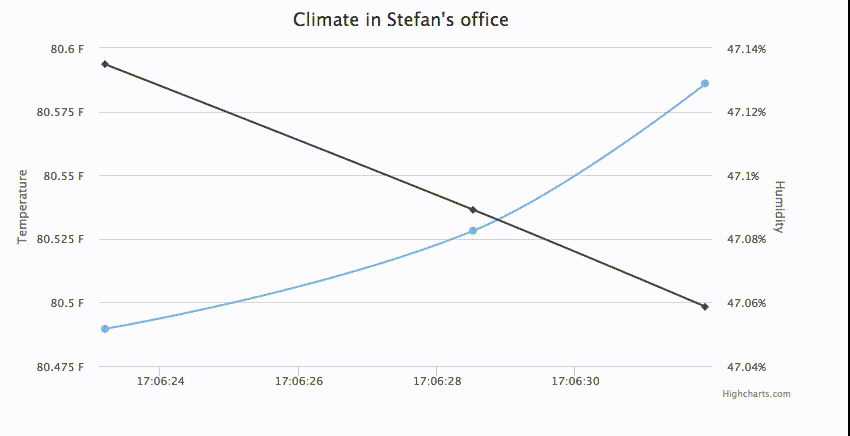
Very impressive how quick that was to wire up. That's in part because everything is JavaScript, so writing the code was trivial. There's on big downside, though. Unlike the Arduino, the Tessel just isn't ready for prime time yet. It seems like the biggest problem is the Wifi chip that causes the whole system to hang and stop responding after a while. There are lots of people on the Tessel forums who seem to have that same issue. I've tried many of the suggestions to no avail. I think most applications of microcontrollers in general depend on them being reliably up for days if not months at a time and it seems like Tessel can't deliver on that front yet. I'm hoping they figure out those issues for the Tessel 2, which I nevertheless preordered.
The code for this is up on github:
https://github.com/stefanbaumg/tessel-climate