Custom Login Buttons in Meteor
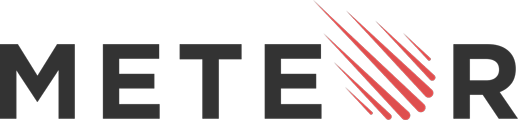
Meteor comes with two great packages that make it easy to display login buttons to the user: accounts-ui and accounts-ui-unstyled. As you may have guessed, the unstyled version gives you a pretty bare bones version of the login buttons. But what if that bare bones version isn't quite enough because you want to have full control over your markup as well as the login flow?
Fortunately, Meteor also comes with some built-in methods that make building your own buttons a snap, namely Meteor.loginWith...().
What I wanted for my project was a simple button for logging in with Google that looks like this:
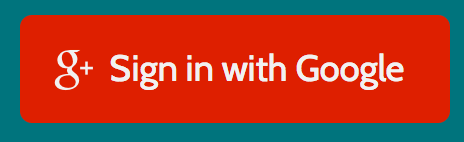
During the login, I wanted to request access to the user's Picasa/Google+ photos as well as offline access.
The template is very straightforward:
<template name="loginButtonsBig">
<a id="loginGoogle" class="btn btn-default btn-lg btn-login google" href="#">
<i class="fa fa-google-plus fa-fw"></i> Sign in with Google
</a>
</template>
```
And all we need is a simple helper method to make this all work:
````prettyprint lang-html
Template.loginButtonsBig.events({
'click a#loginGoogle': function(e, t) {
e.preventDefault();
Meteor.loginWithGoogle({
requestPermissions: ['https://picasaweb.google.com/data/'],
requestOfflineToken: 'true'
}, Router.go('user'));
}
});
```
As you can see, there's a callback which I use to execute an iron router route.
The same kind of code will also work with the other login providers that are supported by Meteor such as Meteor.loginWithTwitter(), etc.